Customers API
The Customers API is changing and will require existing developers to migrate by March 5th! To learn what's changing, refer to the release notes.
The Customers API enables you to directly pass the KYC information to Bridge. This endpoint enables you to control the UI independently and handle all of the back and forth with customers, regardless of whether they are individuals or businesses.
To determine whether a customer is able to use the platform, Bridge monitors the customer's kyc_status
and the status
of each requested endorsement, e.g.base
endorsement for access to US rails.
If Bridge is able to validate the information, the customer will receive KYC approval and be granted the requested endorsement(s) to use Bridge's platform.
If certain information was invalid or missing, the customer object will return the appropriate KYC status, endorsement status, and endorsement requirements.
After obtaining a signed_agreement_id
(details here: Terms of Service), you can now use the Customers API to create customers as follows.
Individual customer creation
curl --location --request POST 'https://api.bridge.xyz/v0/customers' \
--header 'Content-Type: application/json' \
--header 'Api-Key: <API Key>' \
--header 'Idempotency-Key: <generate a uuid>' \
--data-raw '{
"type": "individual",
"first_name": "John",
"last_name": "Doe",
"email": "email@example.com",
"address": {
"street_line_1": "123 Main St",
"city": "New York City",
"subdivision": "New York",
"postal_code": "10001",
"country": "USA"
},
"birth_date": "2007-01-01",
"signed_agreement_id": "d536a227-06d3-4de1-acd3-8b5131730480",
"identifying_information": [
{
"type": "ssn",
"issuing_country": "usa",
"number": "xxx-xx-xxxx"
},
{
"type": "drivers_license",
"issuing_country": "usa",
"number": "xxxxxxxxxxxxx",
"image_front": "data:image/jpg;base64,...",
"image_back": "data:image/jpg;base64,..."
}
]
}
curl --location --request POST 'https://api.bridge.xyz/v0/customers' \
--header 'Content-Type: application/json' \
--header 'Api-Key: <API Key>' \
--header 'Idempotency-Key: <generate a uuid>' \
--data-raw '{
"type": "individual",
"first_name": "John",
"last_name": "Doe",
"email": "johndoe@johndoe.com",
"phone": "+12223334444",
"address": {
"street_line_1": "Juncal 2091",
"street_line_2": "B1648",
"city": "Tigre",
"state": "B", // ISO 3166-2 Subdivision code without the country prefix
"postal_code": "B7000",
"country": "ARG" // ISO 3166-1 alpha3 Country code
},
"signed_agreement_id": <signed_agreement_id from above>,
"birth_date": "1989-09-09",
"tax_identification_number": "111111111",
"gov_id_country": "USA",
"gov_id_image_front" <data-uri>,
"gov_id_image_back" <data-uri> (optional),
"proof_of_address_document" <data-uri> (optional)
}'
"type": "individual",
"first_name": "John",
"last_name": "Doe",
"email": "email@example.com",
"address": {
"street_line_1": "Juncal 2091",
"street_line_2": "B1648",
"city": "Tigre",
"subdivision": "B", // ISO 3166-2 Subdivision code without the country prefix
"postal_code": "B7000",
"country": "ARG" // ISO 3166-1 alpha3 Country code
},
"birth_date": "2007-01-01",
"signed_agreement_id": "d536a227-06d3-4de1-acd3-8b5131730480",
"employment_status": "employed",
"expected_monthly_payments": "5000_9999",
"acting_as_intermediary": "no",
"most_recent_occupation": "291291",
"account_purpose": "purchase_goods_and_services",
"account_purpose_other": null,
"source_of_funds": "salary",
"identifying_information": [
{
"type": "passport",
"issuing_country": "arg",
"number": "xxxxxxxxxxxxx",
"image_front": "data:image/jpg;base64,...",
"image_back": "data:image/jpg;base64,..."
}
]
}
curl --location --request POST 'https://api.bridge.xyz/v0/customers' \
--header 'Content-Type: application/json' \
--header 'Api-Key: <API Key>' \
--header 'Idempotency-Key: <generate a uuid>' \
--data-raw '{
"type": "individual",
"first_name": "John",
"last_name": "Doe",
"email": "email@example.com",
"address": {
"street_line_1": "80 Queens Road",
"street_line_2": "Suite 3B",
"city": "Manchester",
"subdivision": "MAN", // ISO 3166-2 Subdivision code without the country prefix
"postal_code": "M1 1AE",
"country": "GBR" // ISO 3166-1 alpha3 Country code
},
"birth_date": "2007-01-01",
"signed_agreement_id": "d536a227-06d3-4de1-acd3-8b5131730480",
"identifying_information": [
{
"type": "passport",
"issuing_country": "gbr",
"number": "xxxxxxxxxxxxx",
"image_front": "data:image/jpg;base64,...",
}
],
"documents": [
{
"purposes": [
"proof_of_address"
],
"file": "data:image/jpg;base64,..."
}
]
}
Note: proof_of_address_document
is required for individual customers from European Economic Area (EEA)
, or customers who wish to use SEPA/Euro services offered by Bridge. Please refer to SEPA/Euro Transactions page for details.
For international customers, submit an ISO 3166-1 alpha-3 country code and an ISO 3166-2 subdivision code (minus the country prefix) for the state. So for the above example, GB-MAN
is the code, but just MAN
is submitted.
Business customer creation
curl --location --request POST 'https://api.bridge.xyz/v0/customers' \
--header 'Content-Type: application/json' \
--header 'Api-Key: <API Key>' \
--header 'Idempotency-Key: <generate a uuid>' \
--data-raw '{
"type": "business",
"registered_address": {
street_line_1: "123 Main St",
city: "New York City",
subdivision: "New York",
postal_code: "10001",
country: "USA",
},
"business_type": "corporation",
"business_industry": "1153",
"compliance_screening_explanation": "Ut similique dolores quo.",
"business_description": "A business",
"email": "team@business.co",
"is_dao": false,
"is_high_risk": false,
"business_legal_name": "My Business",
"has_material_intermediary_ownership": false,
"service_usage_description": "Description",
"signed_agreement_id": "7262662f-6622-46d1-9ac1-0e840d78598f",
"estimated_annual_revenue_usd": "250000000_plus",
"expected_monthly_payments_usd": 101307,
"operates_in_prohibited_countries": "no",
"account_purpose": "receive_payments_for_goods_and_services",
"account_purpose_other": null,
"high_risk_activities": [
"none_of_the_above"
],
"source_of_funds": "business_loans",
"source_of_funds_description": "Minima aperiam cum aut.",
"conducts_money_services": true,
"conducts_money_services_using_bridge": true,
identifying_information: [
{
type: "ein",
issuing_country: "usa",
number: "xxx-xx-xxxx",
},
],
documents: [
{
purposes: ["statement_of_funds"],
file: "data:image/jpg;base64,...",
},
{
purposes: ["flow_of_funds"],
file: "data:image/jpg;base64,...",
},
{
purposes: ["ownership_document", "proof_of_address_document"],
file: "data:image/jpg;base64,...",
},
{
purposes: ["formation_document"],
file: "data:image/jpg;base64,...",
},
],
"ultimate_beneficial_owners": [
{
"first_name": "John",
"last_name": "Doe",
"birth_date": "1990-01-01",
"email": "john.doe@gmail.com",
"phone": "1234567890",
identifying_information: [
{
type: "ssn",
issuing_country: "usa",
number: "xxx-xx-xxxx",
},
{
type: "drivers_license",
issuing_country: "usa",
number: "xxxxxxxxxxxxx",
image_front: "data:image/jpg;base64,...",
image_back: "data:image/jpg;base64,...",
},
],
documents: [
{
purposes: ["proof_of_address"],
file: "data:image/jpg;base64,...",
},
],
"address": {
"street_line_1": "1482 Brock Walk",
"street_line_2": "Suite 927",
"city": "Lake Shirley",
"subdivision": null,
"postal_code": null,
"country": "BVT"
},
"has_ownership": true,
"ownership_percentage": "25",
"is_director": true,
"has_control": true,
"is_signer": true,
"title": "CEO",
"relationship_established_at": "2015-01-01",
}
],
"primary_website": "http://google.com"
}
Customer status
Upon customer creation, Bridge will review the submitted customer information and return statuses for both overall KYC (kyc_status
) as well as a status for each requested endorsement (e.g. base
, the endorsement for US rails, will have its own status
). Bridge recommends using both kyc_status
and endorsement status
to determine the customer's ability to use the Bridge platform.
KYC status
Upon customer creation, Bridge will review all KYCs for the customer and return a status
field that denotes the KYC status (as applicable) for the user (example KYC statuses include not_started
, active
, or rejected
). The average decision time for KYC is typically less than one minute. If a manual review is required for KYC, the decision may take until next business day. KYB reviews typically happen same business day, up to next business day.
A sample response would look like:
// 201 CREATED
{
"id": "cust_john_uuid",
"first_name": "John",
"kyc_status": "not_started",
// ...
"requirements_due": [
"external_account" // Customer needs to register their bank account with Bridge
],
"created_at": "Thu, 04 May 2023 15:40:40.832827000 UTC +00:00",
"updated_at": "Thu, 04 May 2023 15:40:40.832827000 UTC +00:00"
}
While KYC generally works quickly, there are edge cases that are important to account for. Here are a few examples of how the KYC status of a customer could progress:
not_started
->active
- This is our happy path for individual customers. The customer information was submitted, and quickly passed via automated checks within seconds.
not_started
->under_review
->approved/rejected
- All business customers and some individual customers may require the Bridge Compliance team to do due diligence of vetting customers manually before the reach a terminal status.
not_started
->rejected
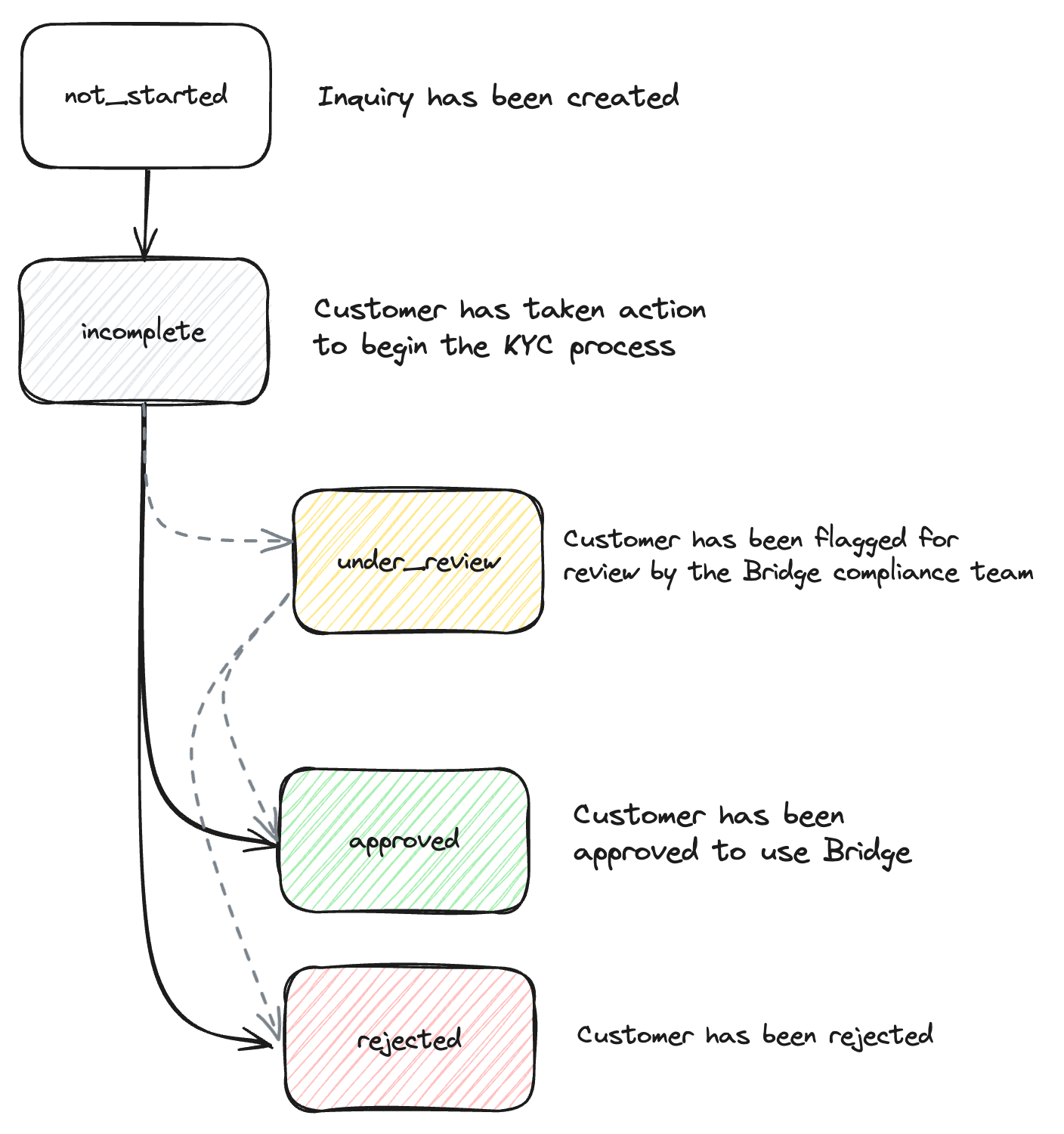
KYC rejection
Sometimes, your customer could immediately move from not_started to rejected and skip under_review entirely. The primary reason this happens is when the tax identification number is entered incorrectly. Without a valid tax identification number, Bridge is unable to verify any of the other details you have submitted for your customer (birth date, address, name, or business details).
In this scenario, the customer starts off not_started
, but after attempting to verify submitted information, there was an issue detected with the submission. It is possible to move directly to rejected
and skip under_review
entirely. In the rejected
scenario, fetching the customer via [GET /customers/:id](https://apidocs.bridge.xyz/reference/get_customers-customerid)
 would result in something like this:
{
"id": "d99a4ee8-03a8-48a6-9cbf-7746a3ce1050",
"email": "john@doe.com",
"first_name": "John",
"last_name": "Doe",
"status": "rejected",
"type": "individual",
"created_at": "2024-02-19T17:16:20.561Z",
"website_url": null,
"persona_idv_link": "...",
"tos_link": "...",
"tos_complete": false,
"persona_inquiry_type": "gov_id_db",
"rejection_reasons": [
{
"developer_reason": "Identity cannot be verified agains third-party databases",
"reason": "Your information could not be verified",
"created_at": "2024-02-19T19:01:59.529Z"
}
]
}
You can see that the customer's KYC status is rejected
 and that there are two fields shared with rejection reasons:
developer_reason
is meant to be used by developers for internal purposes only. This field can contain sensitive information intended for only the developer and is provided to help with troubleshooting potential issues or protecting against potential abuse.reason
can be shared by a developer directly with their customers.
For reference, see our page on Rejection Reasons for developer_reason
and reason
mappings for KYC rejection reasons.
Updating rejected customers
If a customer has had their KYC attempt rejected, their information can be updated at PUT /customers/:id
. This is not applicable to customers who have already had their KYC approved. This endpoint accepts the same shape of data as the creation endpoint.
Notes:
- Even if information was submitted in the first attempt and has not changed, it must still be submitted in the update.
- Updating the customer object won't clear their rejection reasons from earlier attempts, but that won't prevent them passing KYC.
Supported Government ID's by Country
Y = Yes (Supported) | * (Requires backside )
Country code | Country | Subdivision code | Subdivision | Passport extraction | Passport verification | Driver License extraction | Driver License verification | National ID extraction | National ID verification | Other extraction | Other verification | (Requires backside: *) |
---|---|---|---|---|---|---|---|---|---|---|---|---|
AD | Andorra | y | y | y | y | |||||||
AE | United Arab Emirates | y | y | y | y | y* | y | Residency permit* | Residency permit | |||
AF | Afghanistan | y | y | |||||||||
AG | Antigua and Barbuda | y | y | |||||||||
AI | Anguilla | y | y | |||||||||
AL | Albania | y | y | y | y | y* | y | |||||
AM | Armenia | y | y | y | y | y* | y | |||||
AO | Angola | y | y | y | y | y* | y | |||||
AR | Argentina | y | y | y | y | y | y | |||||
AS | American Samoa | y | y | y | y | y | y | |||||
AT | Austria | y | y | y | y | y | y | Residency permit* | Residency permit | |||
AU | Australia | y | y | y* | y | y* | y | Keypass ID, Residency permit* | Keypass ID, Residency permit | |||
AZ | Azerbaijan | y | y | y* | y | y* | y | Residency permit* | Residency permit | |||
BA | Bosnia and Herzegovina | y | y | y | y | y* | y | |||||
BB | Barbados | y | y | y | y | |||||||
BD | Bangladesh | y | y | y | y | y* | y | |||||
BE | Belgium | y | y | y | y | y* | y | Residency permit* | Residency permit | |||
BF | Burkina Faso | y | y | |||||||||
BG | Bulgaria | y | y | y | y | y | y | Residency permit* | Residency permit | |||
BH | Bahrain | y | y | y* | y | |||||||
BI | Burundi | y | y | |||||||||
BJ | Benin | y | y | y | y | y | y | |||||
BM | Bermuda | y | y | y | y | Voter ID | Voter ID | |||||
BN | Brunei Darussalam | y | y | y | y | y* | y | Residency permit* | Residency permit | |||
BO | Bolivia (Plurinational State of) | y | y | y | y | y* | y | |||||
BR | Brazil | y | y | y* | y | y* | y | Residency permit* | Residency permit | |||
BS | Bahamas | y | y | y | y | Work permit* | Work permit | |||||
BT | Bhutan | y | y | |||||||||
BW | Botswana | y | y | y | y | y* | y | |||||
BY | Belarus | y | y | y | y | y | y | |||||
BZ | Belize | y | y | |||||||||
CA | Canada | y | y | 13 | 13 | 12 | 12 | Citizen certificate, Healthcare Insurance card, Passport card, Permanent resident card*, Tribal ID | Citizen certificate, Healthcare Insurance card, Passport card, Permanent resident card, Tribal ID | |||
CA | Canada | CA-AB | Alberta | y | y | y | y | |||||
CA | Canada | CA-BC | British Columbia | y | y | y | y | |||||
CA | Canada | CA-MB | Manitoba | y | y | y | y | |||||
CA | Canada | CA-NB | New Brunswick | y | y | y | y | |||||
CA | Canada | CA-NL | Newfoundland and Labrador | y | y | y | y | |||||
CA | Canada | CA-NS | Nova Scotia | y | y | y | y | |||||
CA | Canada | CA-ON | Ontario | y | y | y | y | |||||
CA | Canada | CA-PE | Prince Edward Island | y | y | y | y | |||||
CA | Canada | CA-QC | Quebec | y | y | |||||||
CA | Canada | CA-SK | Saskatchewan | y | y | y | y | |||||
CA | Canada | CA-NT | Northwest Territories | y | y | y | y | |||||
CA | Canada | CA-NU | Nunavut | y | y | y | y | |||||
CA | Canada | CA-YT | Yukon | y | y | y | y | |||||
CD | Congo (Democratic Republic of the) | y | y | Voter ID | Voter ID | |||||||
CF | Central African Republic | y | y | |||||||||
CG | Congo | y | y | y* | y | y* | y | |||||
CH | Switzerland | y | y | y | y | y* | y | Residency permit* | Residency permit | |||
CI | Côte d'Ivoire | y | y | y* | y | y* | y | |||||
CL | Chile | y | y | y | y | y | y | |||||
CM | Cameroon | y | y | y | y | y* | y | |||||
CN | China | y | y | y | y | y* | y | |||||
CO | Colombia | y | y | y* | y | y* | y | Foreigner ID | Foreigner ID | |||
CR | Costa Rica | y | y | y | y | y* | y | |||||
CU | Cuba | y | y | y* | y | |||||||
CV | Cabo Verde | y | y | |||||||||
CW | Curaçao | y | y | y | y | y | y | |||||
CY | Cyprus | y | y | y | y | y* | y | Residency permit* | Residency permit | |||
CZ | Czech Republic | y | y | y | y | y* | y | Residency permit* | Residency permit | |||
DE | Germany | y | y | y | y | y* | y | Residency permit* | Residency permit | |||
DJ | Djibouti | y | y | |||||||||
DK | Denmark | y | y | y | y | Residency permit* | Residency permit | |||||
DM | Dominica | y | y | |||||||||
DO | Dominican Republic | y | y | y* | y | y* | y | |||||
DZ | Algeria | y | y | y* | y | |||||||
EC | Ecuador | y | y | y | y | y* | y | Consular ID | Consular ID | |||
EE | Estonia | y | y | y | y | y* | y | Residency permit* | Residency permit | |||
EG | Egypt | y | y | y | y | y* | y | |||||
ER | Eritrea | y | y | |||||||||
ES | Spain | y | y | y | y | y* | y | Residency permit* | Residency permit | |||
ET | Ethiopia | y | y | y | y | y | y | Residency permit* | Residency permit | |||
FI | Finland | y | y | y | y | y* | y | Residency permit* | Residency permit | |||
FJ | Fiji | y | y | y | y | |||||||
FM | Micronesia (Federated States of) | y | y | |||||||||
FO | Faroe Islands | y | y | y | y | |||||||
FR | France | y | y | y | y | y* | y | Residency permit* | Residency permit | |||
GA | Gabon | y | y | |||||||||
GB | United Kingdom of Great Britain and Northern Ireland | y | y | y | y | y | y | Residency permit*, Voter ID | Residency permit, Voter ID | |||
GD | Grenada | y | y | |||||||||
GE | Georgia | y | y | y | y | y* | y | |||||
GG | Guernsey | y | y | y | y | y | y | |||||
GH | Ghana | y | y | y | y | y* | y | Voter ID | Voter ID | |||
GI | Gibraltar | y | y | y | y | y* | y | |||||
GL | Greenland | y | y | |||||||||
GM | Gambia | y | y | |||||||||
GN | Guinea | y | y | |||||||||
GQ | Equatorial Guinea | y | y | |||||||||
GR | Greece | y | y | y | y | y* | y | Residency permit* | Residency permit | |||
GT | Guatemala | y | y | y* | y | y* | y | Consular ID* | Consular ID | |||
GU | Guam | y | y | y | y | y | y | |||||
GW | Guinea-Bissau | y | y | |||||||||
GY | Guyana | y | y | |||||||||
HK | Hong Kong | y | y | y | y | |||||||
HN | Honduras | y | y | y* | y | y | y | Consular ID | Consular ID | |||
HR | Croatia | y | y | y* | y | y* | y | Residency permit* | Residency permit | |||
HT | Haiti | y | y | |||||||||
HU | Hungary | y | y | y | y | y* | y | Residency permit* | Residency permit | |||
ID | Indonesia | y | y | y | y | y | y | |||||
IE | Ireland | y | y | y | y | y* | y | Passport card, Residency permit | Passport card, Residency permit | |||
IL | Israel | y | y | y | y | y* | y | |||||
IM | Isle of Man | y | y | y | y | |||||||
IN | India | y | y | y* | y | y | y | Permanent Account Number (PAN) card, Voter ID* | Permanent Account Number (PAN) card, Voter ID | |||
IQ | Iraq | y | y | y* | y | |||||||
IS | Iceland | y | y | y | y | Residency permit* | Residency permit | |||||
IT | Italy | y | y | y | y | y* | y | Residency permit* | Residency permit | |||
JE | Jersey | y | y | y | y | |||||||
JM | Jamaica | y | y | y | y | y | y | Voter ID | Voter ID | |||
JO | Jordan | y | y | y | y | y* | y | |||||
JP | Japan | y | y | y | y | My Number card, Residency permit*, Visa | My Number card, Residency permit, Visa | |||||
KE | Kenya | y | y | y | y | y* | y | Residency permit* | Residency permit | |||
KG | Kyrgyzstan | y | y | y | y | y* | y | |||||
KH | Cambodia | y | y | y | y | |||||||
KI | Kiribati | y | y | |||||||||
KM | Comoros | y | y | |||||||||
KN | Saint Kitts and Nevis | y | y | |||||||||
KR | Korea (Republic of) | y | y | y | y | y | y | Residency permit* | Residency permit | |||
KW | Kuwait | y | y | y* | y | y | y | |||||
KY | Cayman Islands | y | y | y | y | |||||||
KZ | Kazakhstan | y | y | y | y | y* | y | |||||
LA | Lao People's Democratic Republic | y | y | y | y | |||||||
LB | Lebanon | y | y | y | y | y | y | |||||
LC | Saint Lucia | y | y | |||||||||
LI | Liechtenstein | y | y | y | y | y* | y | Residency permit* | Residency permit | |||
LK | Sri Lanka | y | y | y | y | y* | y | |||||
LR | Liberia | y | y | |||||||||
LS | Lesotho | y | y | |||||||||
LT | Lithuania | y | y | y | y | y* | y | Residency permit* | Residency permit | |||
LU | Luxembourg | y | y | y | y | y* | y | Residency permit* | Residency permit | |||
LV | Latvia | y | y | y | y | y* | y | Residency permit* | Residency permit | |||
LY | Libya | y | y | |||||||||
MA | Morocco | y | y | y* | y | y* | y | |||||
MC | Monaco | y | y | y* | y | Residency permit* | Residency permit | |||||
MD | Moldova (Republic of) | y | y | y | y | y* | y | Residency permit* | Residency permit | |||
ME | Montenegro | y | y | y | y | y* | y | |||||
MF | Saint Martin (French part) | y | y | y | y | |||||||
MG | Madagascar | y | y | |||||||||
MH | Marshall Islands | y | y | |||||||||
MK | North Macedonia | y | y | y | y | y* | y | Residency permit* | Residency permit | |||
ML | Mali | y | y | |||||||||
MM | Myanmar | y | y | y | y | y* | y | |||||
MN | Mongolia | y | y | y* | y | y* | y | |||||
MO | Macao | y | y | Residency permit* | Residency permit | |||||||
MR | Mauritania | y | y | |||||||||
MS | Montserrat | y | y | |||||||||
MT | Malta | y | y | y | y | y* | y | Residency permit* | Residency permit | |||
MU | Mauritius | y | y | y* | y | |||||||
MV | Maldives | y | y | |||||||||
MW | Malawi | y | y | y | y | |||||||
MX | Mexico | y | y | 32 | 32 | Consular ID, Residency permit, Voter ID* | Consular ID, Residency permit, Voter ID | |||||
MX | Mexico | MX-AG | y | y | ||||||||
MX | Mexico | MX-BC | y | y | ||||||||
MX | Mexico | MX-BS | y | y | ||||||||
MX | Mexico | MX-CM | y | y | ||||||||
MX | Mexico | MX-CS | y | y | ||||||||
MX | Mexico | MX-CH | y | y | ||||||||
MX | Mexico | MX-CO | y | y | ||||||||
MX | Mexico | MX-CL | y | y | ||||||||
MX | Mexico | MX-DF | y | y | ||||||||
MX | Mexico | MX-DG | y | y | ||||||||
MX | Mexico | MX-GT | y | y | ||||||||
MX | Mexico | MX-GR | y | y | ||||||||
MX | Mexico | MX-HG | y | y | ||||||||
MX | Mexico | MX-JA | y | y | ||||||||
MX | Mexico | MX-EM | y | y | ||||||||
MX | Mexico | MX-MI | y | y | ||||||||
MX | Mexico | MX-MO | y | y | ||||||||
MX | Mexico | MX-NA | y | y | ||||||||
MX | Mexico | MX-NL | y | y | ||||||||
MX | Mexico | MX-OA | y | y | ||||||||
MX | Mexico | MX-PU | y | y | ||||||||
MX | Mexico | MX-QT | y | y | ||||||||
MX | Mexico | MX-QR | y | y | ||||||||
MX | Mexico | MX-SL | y | y | ||||||||
MX | Mexico | MX-SI | y | y | ||||||||
MX | Mexico | MX-SO | y | y | ||||||||
MX | Mexico | MX-TB | y | y | ||||||||
MX | Mexico | MX-TM | y | y | ||||||||
MX | Mexico | MX-TL | y | y | ||||||||
MX | Mexico | MX-VE | y | y | ||||||||
MX | Mexico | MX-YU | y | y | ||||||||
MX | Mexico | MX-ZA | y | y | ||||||||
MY | Malaysia | y | y | y | y | y* | y | |||||
MZ | Mozambique | y | y | y* | y | |||||||
NA | Namibia | y | y | y* | y | |||||||
NE | Niger | y | y | |||||||||
NG | Nigeria | y | y | y | y | y* | y | Voter ID | Voter ID | |||
NI | Nicaragua | y | y | y | y | Voter ID | Voter ID | |||||
NL | Netherlands | y | y | y* | y | y* | y | Residency permit* | Residency permit | |||
NO | Norway | y | y | y | y | y* | y | Residency permit* | Residency permit | |||
NP | Nepal | y | y | y | y | y | y | Citizen certificate | Citizen certificate | |||
NR | Nauru | y | y | |||||||||
NZ | New Zealand | y | y | y | y | y | y | |||||
OM | Oman | y | y | y* | y | y* | y | Residency permit* | Residency permit | |||
PA | Panama | y | y | y | y | y | y | Permanent resident card | Permanent resident card | |||
PE | Peru | y | y | y* | y | y* | y | Residency permit* | Residency permit | |||
PG | Papua New Guinea | y | y | |||||||||
PH | Philippines | y | y | y | y | y* | y | Healthcare Insurance card, NBI Clearance, Overseas Foreign Worker card (OFW), Postal ID, Social Security System card, United Multi Purpose ID (UMID), Voter ID | Healthcare Insurance card, NBI Clearance, Overseas Foreign Worker card (OFW), Postal ID, Social Security System card, United Multi Purpose ID (UMID), Voter ID | |||
PK | Pakistan | y | y | y* | y | |||||||
PL | Poland | y | y | y | y | y* | y | Residency permit* | Residency permit | |||
PR | Puerto Rico | y | y | y | y | y | y | |||||
PS | Palestine, State of | y | y | |||||||||
PT | Portugal | y | y | y | y | y* | y | Residency permit* | Residency permit | |||
PW | Palau | y | y | |||||||||
PY | Paraguay | y | y | y | y | y* | y | |||||
QA | Qatar | y | y | y | y | y | y | Residency permit* | Residency permit | |||
RO | Romania | y | y | y | y | y | y | Residency permit* | Residency permit | |||
RS | Serbia | y | y | y | y | y* | y | |||||
RU | Russian Federation | y | y | y | y | Internal passport | Internal passport | |||||
RW | Rwanda | y | y | y | y | y | y | |||||
SA | Saudi Arabia | y | y | y | y | y | y | Residency permit* | Residency permit | |||
SB | Solomon Islands | y | y | |||||||||
SC | Seychelles | y | y | y | y | |||||||
SD | Sudan | y | y | |||||||||
SE | Sweden | y | y | y | y | y* | y | Residency permit* | Residency permit | |||
SG | Singapore | y | y | y | y | y* | y | Long-Term pass card, Work permit | Long-Term pass card, Work permit | |||
SI | Slovenia | y | y | y | y | y* | y | Residency permit* | Residency permit | |||
SK | Slovakia | y | y | y | y | y* | y | Residency permit* | Residency permit | |||
SL | Sierra Leone | y | y | |||||||||
SM | San Marino | y | y | y* | y | |||||||
SN | Senegal | y | y | y | y | y | y | |||||
SO | Somalia | y | y | |||||||||
SR | Suriname | y | y | |||||||||
SS | South Sudan | y | y | |||||||||
ST | Sao Tome and Principe | y | y | |||||||||
SV | El Salvador | y | y | y* | y | y* | y | |||||
SZ | eSwatini | y | y | |||||||||
TC | Turks and Caicos Islands | y | y | |||||||||
TD | Chad | y | y | |||||||||
TG | Togo | y | y | |||||||||
TH | Thailand | y | y | y | y | y* | y | |||||
TJ | Tajikistan | y | y | |||||||||
TL | Timor-Leste | y | y | |||||||||
TM | Turkmenistan | y | y | |||||||||
TN | Tunisia | y | y | y | y | y* | y | |||||
TO | Tonga | y | y | |||||||||
TR | Turkey | y | y | y* | y | y* | y | Residency permit* | Residency permit | |||
TT | Trinidad and Tobago | y | y | y | y | y* | y | |||||
TV | Tuvalu | y | y | |||||||||
TW | Taiwan | y | y | y | y | y* | y | Healthcare Insurance card, Residency permit* | Healthcare Insurance card, Residency permit | |||
TZ | Tanzania, United Republic of | y | y | y | y | y | y | Voter ID | Voter ID | |||
UA | Ukraine | y | y | y | y | Passport card, Residency permit | Passport card, Residency permit | |||||
UG | Uganda | y | y | y | y | y | y | |||||
US | United States of America | y | y | 51 | 51 | 51 | 51 | Passport card, Permanent resident card, Travel Document, Visa, Work permit* | Passport card, Permanent resident card, Travel Document, Visa, Work permit | |||
US | United States of America | US-AL | Alabama | y | y | y | y | |||||
US | United States of America | US-AK | Alaska | y | y | y | y | |||||
US | United States of America | US-AZ | Arizona | y | y | y | y | |||||
US | United States of America | US-AR | Arkansas | y | y | y | y | |||||
US | United States of America | US-CA | California | y | y | y | y | |||||
US | United States of America | US-CO | Colorado | y | y | y | y | |||||
US | United States of America | US-CT | Connecticut | y | y | y | y | |||||
US | United States of America | US-DC | District of Columbia | y | y | y | y | |||||
US | United States of America | US-DE | Delaware | y | y | y | y | |||||
US | United States of America | US-FL | Florida | y | y | y | y | |||||
US | United States of America | US-GA | Georgia | y | y | y | y | |||||
US | United States of America | US-HI | Hawaii | y | y | y | y | |||||
US | United States of America | US-ID | Idaho | y | y | y | y | |||||
US | United States of America | US-IL | Illinois | y | y | y | y | |||||
US | United States of America | US-IN | Indiana | y | y | y | y | |||||
US | United States of America | US-IA | Iowa | y | y | y | y | |||||
US | United States of America | US-KS | Kansas | y | y | y | y | |||||
US | United States of America | US-KY | Kentucky | y | y | y | y | |||||
US | United States of America | US-LA | Louisiana | y | y | y | y | |||||
US | United States of America | US-ME | Maine | y | y | y | y | |||||
US | United States of America | US-MD | Maryland | y | y | y | y | |||||
US | United States of America | US-MA | Massachusetts | y | y | y | y | |||||
US | United States of America | US-MI | Michigan | y | y | y | y | |||||
US | United States of America | US-MN | Minnesota | y | y | y | y | |||||
US | United States of America | US-MS | Mississippi | y | y | y | y | |||||
US | United States of America | US-MO | Missouri | y | y | y | y | |||||
US | United States of America | US-MT | Montana | y | y | y | y | |||||
US | United States of America | US-NE | Nebraska | y | y | y | y | |||||
US | United States of America | US-NV | Nevada | y | y | y | y | |||||
US | United States of America | US-NH | New Hampshire | y | y | y | y | |||||
US | United States of America | US-NJ | New Jersey | y | y | y | y | |||||
US | United States of America | US-NM | New Mexico | y | y | y | y | |||||
US | United States of America | US-NY | New York | y | y | y | y | |||||
US | United States of America | US-NC | North Carolina | y | y | y | y | |||||
US | United States of America | US-ND | North Dakota | y | y | y | y | |||||
US | United States of America | US-OH | Ohio | y | y | y | y | |||||
US | United States of America | US-OK | Oklahoma | y | y | y | y | |||||
US | United States of America | US-OR | Oregon | y | y | y | y | |||||
US | United States of America | US-PA | Pennsylvania | y | y | y | y | |||||
US | United States of America | US-RI | Rhode Island | y | y | y | y | |||||
US | United States of America | US-SC | South Carolina | y | y | y | y | |||||
US | United States of America | US-SD | South Dakota | y | y | y | y | |||||
US | United States of America | US-TN | Tennessee | y | y | y | y | |||||
US | United States of America | US-TX | Texas | y | y | y | y | |||||
US | United States of America | US-UT | Utah | y | y | y | y | |||||
US | United States of America | US-VT | Vermont | y | y | y | y | |||||
US | United States of America | US-VA | Virginia | y | y | y | y | |||||
US | United States of America | US-WA | Washington | y | y | y | y | |||||
US | United States of America | US-WV | West Virginia | y | y | y | y | |||||
US | United States of America | US-WI | Wisconsin | y | y | y | y | |||||
US | United States of America | US-WY | Wyoming | y | y | y | y | |||||
UY | Uruguay | y | y | y | y | y* | y | |||||
UZ | Uzbekistan | y | y | y | y | y | y | |||||
VC | Saint Vincent and the Grenadines | y | y | |||||||||
VE | Venezuela (Bolivarian Republic of) | y | y | y | y | y | y | |||||
VI | Virgin Islands (U.S.) | y | y | y | y | y | y | |||||
VN | Viet Nam | y | y | y | y | y | y | |||||
VU | Vanuatu | y | y | |||||||||
WS | Samoa | y | y | |||||||||
XK | Kosovo | y | y | y | y | y* | y | Residency permit* | Residency permit | |||
YE | Yemen | y | y | y | y | |||||||
ZA | South Africa | y | y | y | y | y | y | |||||
ZM | Zambia | y | y | |||||||||
ZW | Zimbabwe | y | y | y | y | y | y |
Updated 24 days ago